What is View Engine in ASP NET MVC?
View Engine:
In ASP.NET MVC, a view engine is merely a class that implements a fixed interface – The IViewEngine interface. Each application can have one or more view engines.
Structure and Behavior of a view engine:
The view engine is the component that physically builds the HTML output for the browser. The view engine engages for each request that returns HTML, and it prepares its output by mixing a template for the view and any data the controller passes in. The template is expressed in an engine-specific markup language, the data is passed packaged in dictionaries or strongly typed objects.
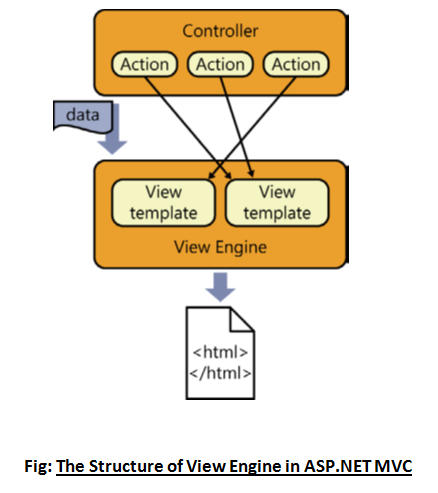
public static class ViewEngines { private static readonly ViewEngineCollection _engines = new ViewEngineCollection { new WebFormViewEngine(), new RazorViewEngine() }; public static ViewEngineCollection Engines { get { return _engines; } } }
Anatomy of a view engine:
A view engine is a class that implements the IViewEngine interface. The contract of the interface says it’s all about the services the engine is expected to provide: the engine is responsible for retrieving a (partial) view object on behalf of the ASP.NET MVC infrastructure. A view object represents the container for any information that is needed to build a real HTML response in ASP.NET MVC. Here are the interface members:
public interface IViewEngine { ViewEngineResult FindPartialView( ControllerContext controllerContext, String partialViewName, Boolean useCache); ViewEngineResult FindView( ControllerContext controllerContext, String viewName, String masterName, Boolean useCache); void ReleaseView( ControllerContext controllerContext, IView view); }